So I want to share one basic technique for counting resources inserted into a chest with combinators. The fundamental thing about how signals work to remember is that it takes 1 tick for a combinator to process its input and produce an output. With that in mind it's relatively straightforward to construct a counter:
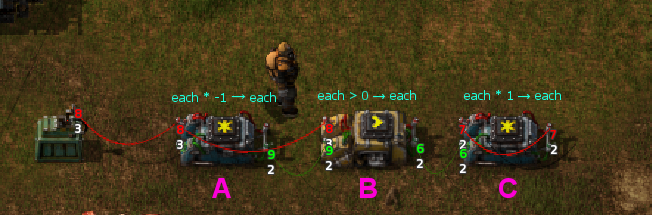
In this setup, the output of C will be a count of the total number of items inserted into the smart chest. If you want to count items removed from a chest, change the condition of B to "each < 0". A manual reset can be done by temporarily changing C to "each * 0" -- this is just a very basic example of the concepts.
The way it works is as follows:
- The input of A is the current inventory of the chest. It outputs the negative of that value on green. But because it takes a tick to process, it is always the negative value of the inventory of the previous game tick.
- The input of B is the current inventory of the chest (red) and the negative value of the inventory of the chest from the previous tick (green). It acts to implicitly sum these two values (count t - count t-1) thus producing delta items per tick, then a filter (> 0) so we only monitor increases.
- The input of C is its own output (red) and the delta item count from B (green). Since B's output is per tick, this effectively acts as a running counter by summing C's previous output with B's momentary output on each tick. The output of C is then the count of items inserted. (It's not important that this is each * 1; it just needs to output identity; so each + 0 works just as well, as does each > 0 -> each with a decider [or each < 0 if you're counting resources removed].)
There is an important caveat here: To do this reliably, you need to have this set of combinators per chest. You don't want to chain multiple chests together as input for just one of these. The reason is that you can run into a situation where items are removed from one of the chests on the same tick as items are added to another chest, and in this case, you'll miss counts (one set of these combinators monitors total resource changes per tick, it doesn't have any awareness of how many chests/tanks/etc. comprise the input).
If this thread already exists then, sorry for creating noise. If not, then I hope this clears things up and gives at least a basic starting point for more complex combinator logic.