With that in mind, I want to talk about the process of circuit design. I'm going to do some examples, but this is more about how I got from concept to final working model than the particular problems.
First example: prioritizing fuels. The destination can be either steam engines or trains, but the idea is to set up something to regulate what gets burned first: wood, coal, or solid fuel. Wood's pretty much scrap, I always want to burn that first. Solid fuel is renewable, but sometimes oil refining is in high demand for plastics and sulfur. Coal is not renewable, and again needed for plastic and sulfur. If I've got a big surplus of either, I want to burn that first, and default to solid fuel if I have shortages.
Unfortunately, unless I've missed something, you can't use inventory sums for the entire logistics network with combinators, the way you can with smart inserters. So the first stumbling block is that I have to use the amounts in specific containers (a storage tank, a single chest) as proxies for my overall totals.
If I were to solve this in a normal programming language, it might look something like this:
Code: Select all
if wood > 0 then
take wood
else
if petroleum gas > 2000 then
take solid fuel
else
if coal > 2000 then
take coal
else
take solid fuel
Code: Select all
if wood > 0 then
take wood
if wood = 0 and petroleum gas > 2000 then
take solid fuel
if wood = 0 and petroleum gas <= 2000 and coal > 2000 then
take coal
if wood = 0 and petroleum gas <= 2000 and coal <= 2000 then
take solid fuel
Code: Select all
if wood = 0 and (petroleum gas > 2000 or coal <= 2000) then
take solid fuel
First, I create a bunch of deciders to codify all the comparisons I wrote in my procedural code.
Code: Select all
decider have_wood( wood > 0 ) : A = 1
decider have_gas( petroleum gas > 2000 ) : B = 1
decider have_coal( coal > 4000 ) : C = 1
// inversions of the above tests - so I only need to change numbers in one place if I change my mind
decider low_wood( have_wood->A = 0 ) : blue = 1
decider low_gas( have_gas->B = 0 ) : green = 1
decider low_coal( have_coal->C = 0 ): red = 1
Code: Select all
// Wood's easy.
inserter wood_inserter( have_wood->A = 1, allow wood )
Code: Select all
// if wood = 0 and petroleum gas <= 2000 and coal > 2000 then
// take coal
// test wood = 0 and gas <= 2000
multiplier multiplier_1( coal = low_wood->blue * low_gas->green )
// test (wood = 0 and gas < 2000) and coal > 2000
multiplier multiplier_2( coal = multiplier_1->coal * have_coal->C )
inserter coal_inserter( multiplier_2->coal > 0, allow coal )
Code: Select all
// if wood = 0 and (petroleum gas > 2000 or coal <= 2000) then
// take solid fuel
// test gas > 2000 or coal <= 2000
adder adder_1( solid_fuel = have_gas->B + low_coal->red )
// wood = 0 AND (gas > 2000 or coal <= 2000)
multiplier multiplier_3( solid_fuel = low_wood->blue * adder_1->solid_fuel )
inserter solid_inserter( multiplier_3->solid_fuel > 0, allow solid fuel )
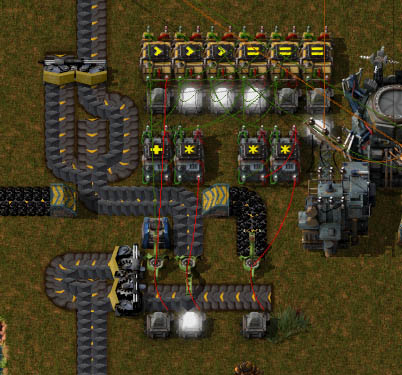
I've added lights below the combinators to make the current state of each clear. I don't have wood, I have both surplus solid fuel and coal, so the circuit chooses solid fuel.