If I can not do it with the attack_parameters, maybe I can create the target marker entity it during on_tick (Turrets do not fire an event when they shoot afaik). Is there some better documentation on attack_parameters and projectiles than the wiki? (since I have a hard time understanding it)
Also, unrelated question: Am I right to ask mod-making questions here or is there a better place?
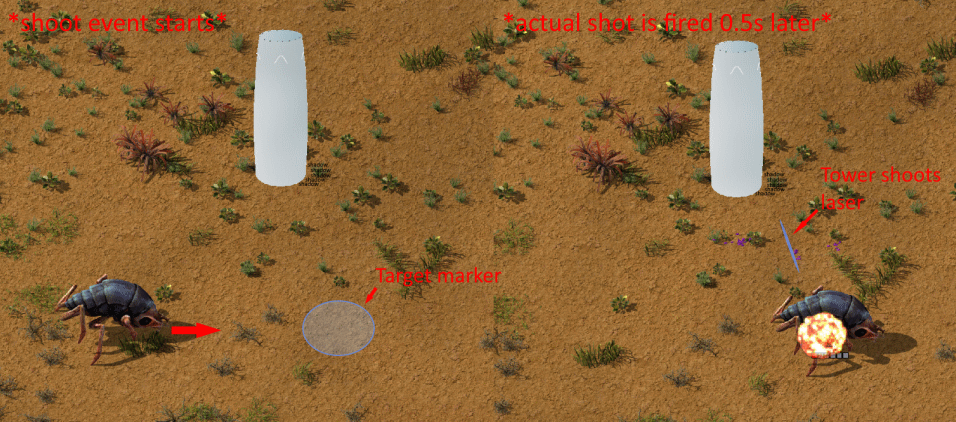