[Resolved] Creating Multiple Entities
[Resolved] Creating Multiple Entities
Hi,
I want to create 100 new enemy entities.
I was wondering if there was a easy way to do this, instead of me physically creating the 100 entities.
If I just want to call the newly created enemies: Unit1 to Unit100.
Is there a way, maybe with deep-copy or something I can use to do this? Then I can just use a reference table for their health and damage or something.
Thanks.
I want to create 100 new enemy entities.
I was wondering if there was a easy way to do this, instead of me physically creating the 100 entities.
If I just want to call the newly created enemies: Unit1 to Unit100.
Is there a way, maybe with deep-copy or something I can use to do this? Then I can just use a reference table for their health and damage or something.
Thanks.
Last edited by TheSAguy on Tue Jul 18, 2017 2:31 pm, edited 3 times in total.
Re: Creating Multiple Entities
You mean create lots of prototypes? Something like
Code: Select all
health = { 5, 10, 20 }
damage = { 7, 15, 25 }
for i = 1, #health do
new_biter = table.deepcopy(data.raw.unit["small-biter"])
new_biter.name = "new-biter-" .. i
new_biter.max_health = health[i]
new_biter.attack_parameters.ammo_type.action.action_delivery.target_effects.damage.amount = damage[i]
data:extend{new_biter}
end
Automatic Belt (and pipe) Planner—Automate yet another aspect of constructing your factory!
Re: Creating Multiple Entities
I think
Code: Select all
local new_biter = ...
Re: Creating Multiple Entities
That really won't make a difference there.
Automatic Belt (and pipe) Planner—Automate yet another aspect of constructing your factory!
Re: Creating Multiple Entities
Thanks guys, I'll give it a try when I'm back from vacation 

- bobingabout
- Smart Inserter
- Posts: 7352
- Joined: Fri May 09, 2014 1:01 pm
- Contact:
Re: Creating Multiple Entities
Going by this they'll all be the size and colour of a small biter, but it's a good way to start.
I'd do things differently, but then the way I do things wouldn't be designed for as many as 100.
And if you are working with as many as 100, I'd suggest replacing those input tables with a formula instead.
I'd do things differently, but then the way I do things wouldn't be designed for as many as 100.
And if you are working with as many as 100, I'd suggest replacing those input tables with a formula instead.
Re: Creating Multiple Entities
I have something weird happening when I'm trying to do a tint change. (Well, weird for me
)
For some reason, when I do a Random tint, it works, but when applying a specific tint, PER newly created Unit, it does not work.
So, what I'm saying is that:
The below code words, creating a random tint:
but the below does not work:
The above is just an example, I actually have a 100 iterations. Full code below.
Here is a screenshot of the random tint, vs. the tint that goes from a=0 to a=255:
and
with the same result.
I think the same issue is happening with the collision/selection boxes.
All the other stuff seems to work, Health, Scale, Damage.
Code:
Code attached.
Thanks.

For some reason, when I do a Random tint, it works, but when applying a specific tint, PER newly created Unit, it does not work.
So, what I'm saying is that:
The below code words, creating a random tint:
Code: Select all
alien_tint1 = { {r=math.random(255), g=math.random(255), b=math.random(255), a=255}, {r=math.random(255), g=math.random(255), b=math.random(255), a=255}, {r=math.random(255), g=math.random(255), b=math.random(255), a=255}, {r=math.random(255), g=math.random(255), b=math.random(255), a=255}, {r=math.random(255), g=math.random(255), b=math.random(255), a=255}}
Code: Select all
alien_tint1 = { {r=0, g=0, b=0, a=255}, {r=3, g=0, b=0, a=255}, {r=5, g=0, b=0, a=255}, {r=8, g=0, b=0, a=255}, {r=10, g=0, b=0, a=255}, {r=13, g=0, b=0, a=255}}
Here is a screenshot of the random tint, vs. the tint that goes from a=0 to a=255:
Images
I've tried to apply the tint in two ways:
Code: Select all
alien_tint1 = { {r=0, g=0, b=0, a=255}, {r=3, g=0, b=0, a=255}, ..... to 100 }
Code: Select all
alien_tint1 = {
["alien-army-1"] ={r=0, g=0, b=0, a=255},
["alien-army-2"] = {r=3, g=0, b=0, a=255},
["alien-army-3"] = {r=5, g=0, b=0, a=255},
----
["alien-army-100"] = {r=255, g=0, b=0, a=255}
}
I think the same issue is happening with the collision/selection boxes.
All the other stuff seems to work, Health, Scale, Damage.
Code:
Code: Select all
for i = 1, 100 do
alien_army = table.deepcopy(data.raw.unit["base-alien"])
alien_army.name = "alien-army-" .. i
alien_army.collision_box = v_collision_box[i]
alien_army.selection_box = v_selection_box[i]
alien_army.sticker_box = v_collision_box[i]
alien_army.max_health = health[i]
alien_army.corpse = "alien-corpse-" .. i
alien_army.attack_parameters.ammo_type.action.action_delivery.target_effects.damage = damage_amount[i]
--Option 1:
alien_army.attack_parameters.animation = biterattackanimation(alien_scale[i], alien_tint1[i], alien_tint2[i])
alien_army.run_animation = biterrunanimation(alien_scale[i], alien_tint1[i], alien_tint2[i])
--Option2:
--alien_army.attack_parameters.animation = biterattackanimation(alien_scale[i], alien_tint1["alien-army-" .. i], alien_tint2["alien-army-" .. i])
--alien_army.run_animation = biterrunanimation(alien_scale[i], alien_tint1["alien-army-" .. i], alien_tint2["alien-army-" .. i])
data:extend{alien_army}
end
Thanks.
- Attachments
-
- alien-enemy.lua
- Code
- (43.52 KiB) Downloaded 114 times
Re: Creating Multiple Entities
I have an idea - I think I seen something similar with tables in tables.
Try using deepcopy to give actual table copy with tint to data structure.
This would also match your list of things that work and those that don't work. All those that you listed contain tables.
Try using deepcopy to give actual table copy with tint to data structure.
This would also match your list of things that work and those that don't work. All those that you listed contain tables.
Re: Creating Multiple Entities
I do agree it's something to do with tables, in tables...orzelek wrote:I have an idea - I think I seen something similar with tables in tables.
Try using deepcopy to give actual table copy with tint to data structure.
This would also match your list of things that work and those that don't work. All those that you listed contain tables.
Is this what you mean:
Code: Select all
alien_army.attack_parameters.animation = biterattackanimation(alien_scale[i], table.deepcopy(alien_tint1[i]), table.deepcopy(alien_tint2[i]))
alien_army.run_animation = biterrunanimation(alien_scale[i], table.deepcopy(alien_tint1[i]), table.deepcopy(alien_tint2[i]))
Still got this result:
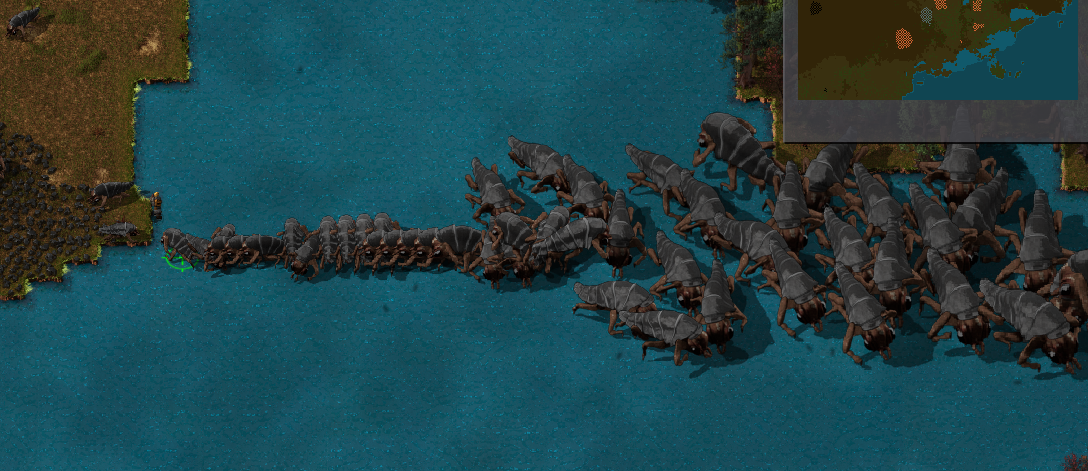
I think the reason the random one works, is because only the first value in the value/table is hit each time. (when dealing with a table in a table) and since the first value is random, it creates a random tint.
Re: Creating Multiple Entities
Can you post the whole mod to make it easier to test stuff?
Re: Creating Multiple Entities
Here is the mod, though I swear it might be working now after not working once the whole weekend...
The Selection and Collision boxes still don't seem to work.
The Selection and Collision boxes still don't seem to work.
- Attachments
-
- Alien_Biters_0.1.0.zip
- Mod
- (15.28 KiB) Downloaded 91 times
Re: Creating Multiple Entities
There must have been a space or something, but is seems like it's working now...
Thanks for checking though.
Thanks for checking though.
- Attachments
-
- Alien_Biters_0.1.0.zip
- Mod
- (13.4 KiB) Downloaded 92 times
Re: Creating Multiple Entities
Thats even more interesting.
Since you have rgba in 0-255 range and game seems to like 0-1 range
Since you have rgba in 0-255 range and game seems to like 0-1 range
