Another example:
You want play Factorio 0.15, but you haven't enough time for it. Let's make all recipes cheaper! Finding in the log file:
2.251 Script data.lua:17: 5 / data.raw.recipe.wood.type = recipe
2.251 Script data.lua:17: 5 / data.raw.recipe.wood.name = wood
2.251 Script data.lua:17: 7 / data.raw.recipe.wood.ingredients.1.1 = raw-wood
2.251 Script data.lua:17: 7 / data.raw.recipe.wood.ingredients.1.2 = 1
2.251 Script data.lua:17: 5 / data.raw.recipe.wood.result = wood
2.251 Script data.lua:17: 5 / data.raw.recipe.wood.result_count = 2
Then our mod have this code:
Code: Select all
for i, recipe in pairs(data.raw.recipe) do
if recipe.type == "recipe" then
if recipe.ingredients then
for i in pairs(recipe.ingredients) do
recipe.ingredients[i][2] = 1
end
if recipe.result then
recipe.result_count = 2
end
end
end
That's all!
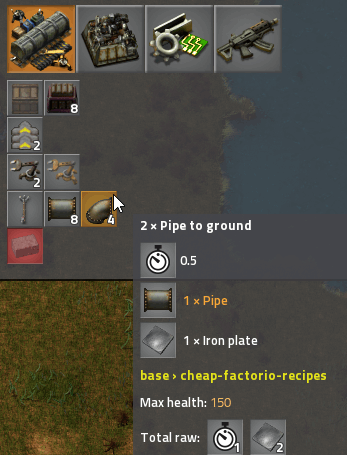
- First try
- 2017-04-26 21_24_14.png (73.73 KiB) Viewed 7192 times
Really? Not all of them. By the 0.15 we have normal and expensive recipes:
1.570 Script data.lua:17: 5 / data.raw.recipe.electric-mining-drill.type = recipe
1.570 Script data.lua:17: 5 / data.raw.recipe.electric-mining-drill.name = electric-mining-drill
1.570 Script data.lua:17: 6 / data.raw.recipe.electric-mining-drill.normal.energy_required = 2
1.570 Script data.lua:17: 8 / data.raw.recipe.electric-mining-drill.normal.ingredients.1.1 = electronic-circuit
1.570 Script data.lua:17: 8 / data.raw.recipe.electric-mining-drill.normal.ingredients.1.2 = 3
1.571 Script data.lua:17: 8 / data.raw.recipe.electric-mining-drill.normal.ingredients.2.1 = iron-gear-wheel
1.571 Script data.lua:17: 8 / data.raw.recipe.electric-mining-drill.normal.ingredients.2.2 = 5
1.571 Script data.lua:17: 8 / data.raw.recipe.electric-mining-drill.normal.ingredients.3.1 = iron-plate
1.571 Script data.lua:17: 8 / data.raw.recipe.electric-mining-drill.normal.ingredients.3.2 = 10
1.571 Script data.lua:17: 6 / data.raw.recipe.electric-mining-drill.normal.result = electric-mining-drill
It means, if we have .normal, then we are need exception:
Code: Select all
for i, recipe in pairs(data.raw.recipe) do
if recipe.type == "recipe" then
if recipe.ingredients then
for i in pairs(recipe.ingredients) do
recipe.ingredients[i][2] = 1
end
if recipe.result then
recipe.result_count = 2
end
elseif recipe.normal.ingredients then -- for 0.15 for normal and expensive recipes
for i in pairs(recipe.normal.ingredients) do
recipe.normal.ingredients[i][2] = 1
end
if recipe.normal.result then
recipe.normal.result_count = 2
end
end
end
end
And now by the normal difficult settings we are have cheap recipes!
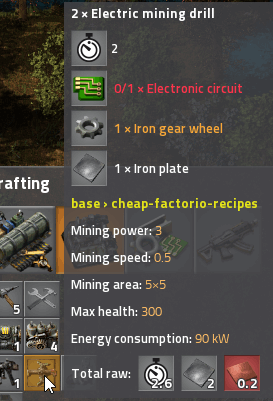
- Second try
- 2017-04-26 21_32_19.png (51.39 KiB) Viewed 7192 times
If you find now recipes with liquids, it's have:
1.542 Script data.lua:17: 7 / data.raw.recipe.plastic-bar.ingredients.1.2 = 1
1.542 Script data.lua:17: 7 / data.raw.recipe.plastic-bar.ingredients.1.type = fluid
1.542 Script data.lua:17: 7 / data.raw.recipe.plastic-bar.ingredients.1.name = petroleum-gas
1.542 Script data.lua:17: 7 / data.raw.recipe.plastic-bar.ingredients.1.amount = 20
1.542 Script data.lua:17: 7 / data.raw.recipe.plastic-bar.ingredients.2.2 = 1
1.542 Script data.lua:17: 7 / data.raw.recipe.plastic-bar.ingredients.2.type = item
1.542 Script data.lua:17: 7 / data.raw.recipe.plastic-bar.ingredients.2.name = coal
1.542 Script data.lua:17: 7 / data.raw.recipe.plastic-bar.ingredients.2.amount = 1
Now you can add another exception and change
.ingredients.1.amount = 20 to
1, and we don't need here
.ingredients.1.2 = 1 anymore.